Changelog
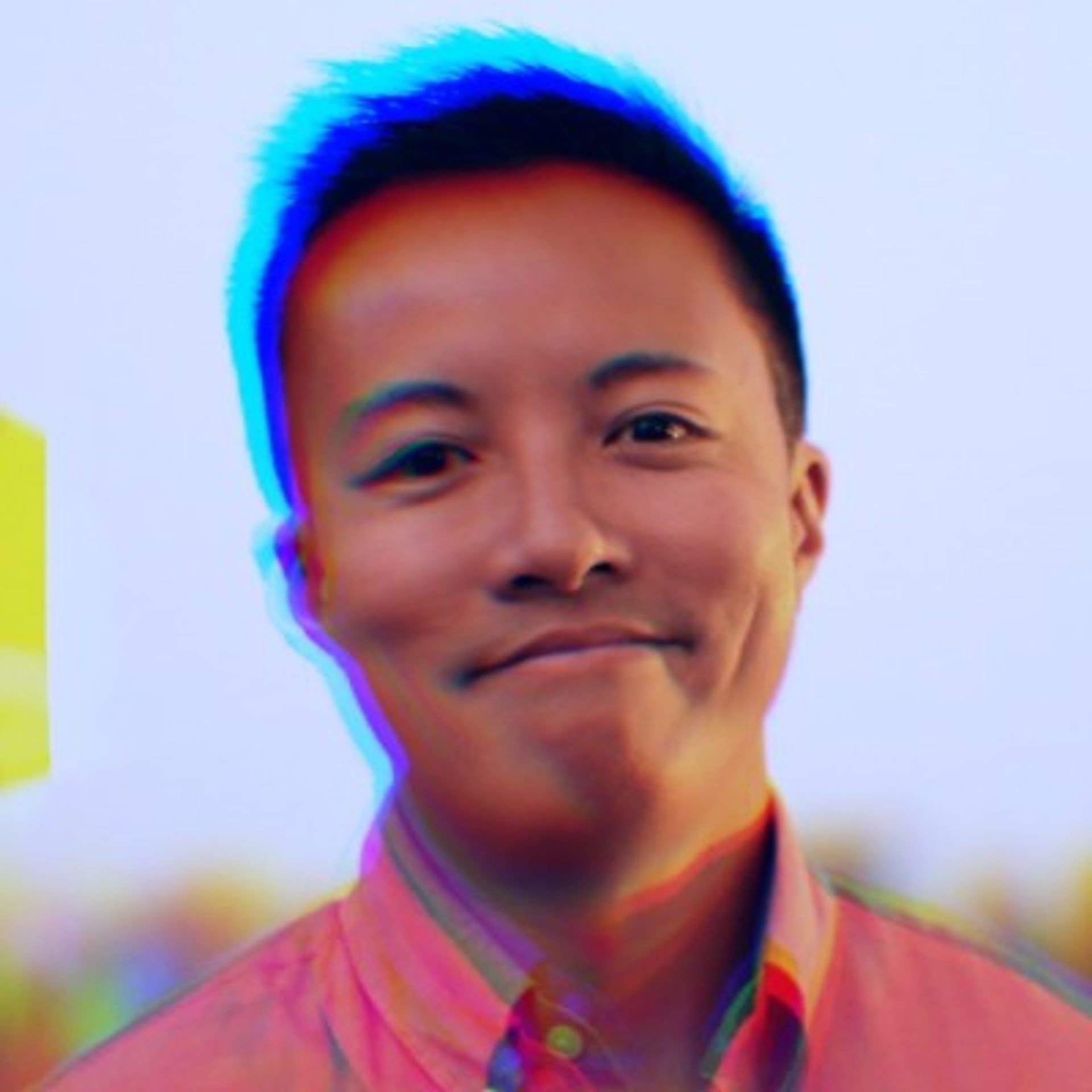
We've made Engine even more reliable in v2.1.20. Here's a sample of nonce management improvements we made:
Transactions can be stuck in mempool for multiple reasons (usually chain-specific). We made improvements to more aggressively retry transactions through including:
- Retries with increasing gas (up to 10x gas).
- Retries after a fixed amount of time, even if the RPC blocks are delayed.
- Configuration to limit the max gas to spend on resends to stay within chain limits.
- Configuration to limit how long to retry a transaction for.
Engine resets the nonce when a wallet runs out of funds to ensure that after the wallet is funded, transactions are sent with correct nonces.
The Retry Failed Transactions now allows retrying any failed transactions including user operations.
Want to force a specific transaction to retry with the same nonce? Use the Retry Transaction (Synchronous) endpoint.
Advanced usage to manage wallet nonces
Most users should rely on Engine to manage and correct wallet nonces. If you want more direct control, the following endpoints allow you to directly manage Engine's wallet nonces. These endpoints are safe to run and intended to "fix" wallets that are stuck. Be warned they may cancel any pending transactions with those nonces.
The Reset Nonces endpoint was updated to optionally allow resetting the nonce state for a single wallet (by default it resets all wallets).
A new Cancel Nonces endpoint allows you to send null transactions with high gas to effectively "cancel" pending transactions using those nonces.
The small team at thirdweb is on a mission to build the most intuitive and complete web3 platform. Our products empower over 70,000 developers each month including Shopify, AWS, Coinbase, Rarible, Animoca Brands, and InfiniGods.
See our open roles. We’d love to work with you!
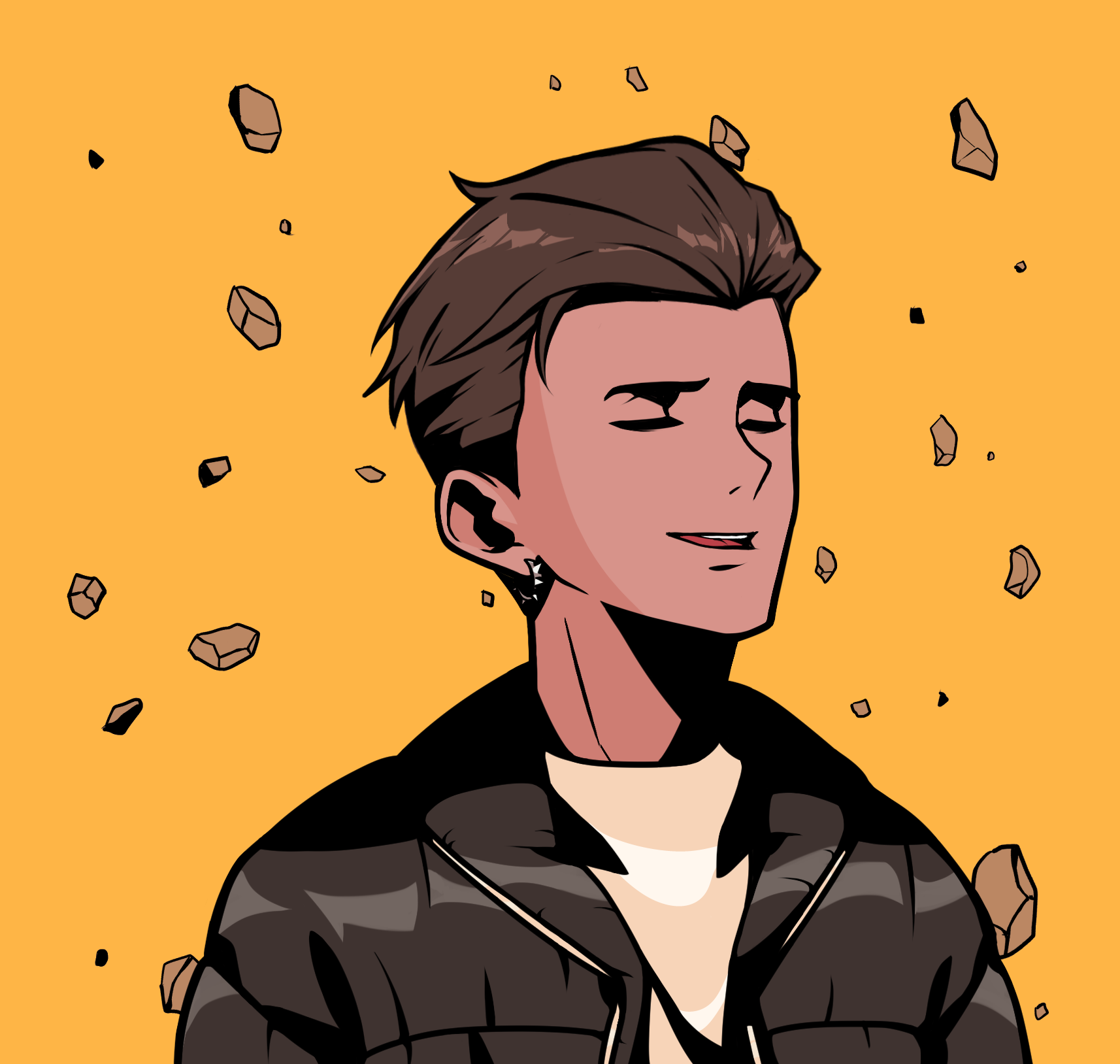
We just released a new package that allows you to use thirdweb's in-app and smart wallets within you wagmi app with minimal effort. Few lines of code to add web2 sign in like google, discord, passkey, etc along with ERC-4337 smart accounts that unlock gasless transactions, batched transactions and session keys.
First, install the thirdweb
and @thirdweb-dev/wagmi-adapter
packages in your wagmi app.
Make sure you're running wagmi 2.14.1 or above.
You probably already have a wagmi config with some connectors, simply add the inAppWalletConnector
to the list, along with the desired options.
Now you can connect users with the desired auth strategy. Options include email, phone, passkey, google, discord, x, telegram, github, and more.
And that's it! The example above connects your users with their google account, creates an ERC4337 smart account with sponsored transactions that you can use with the rest of your wagmi app.
Happy building! 🛠️
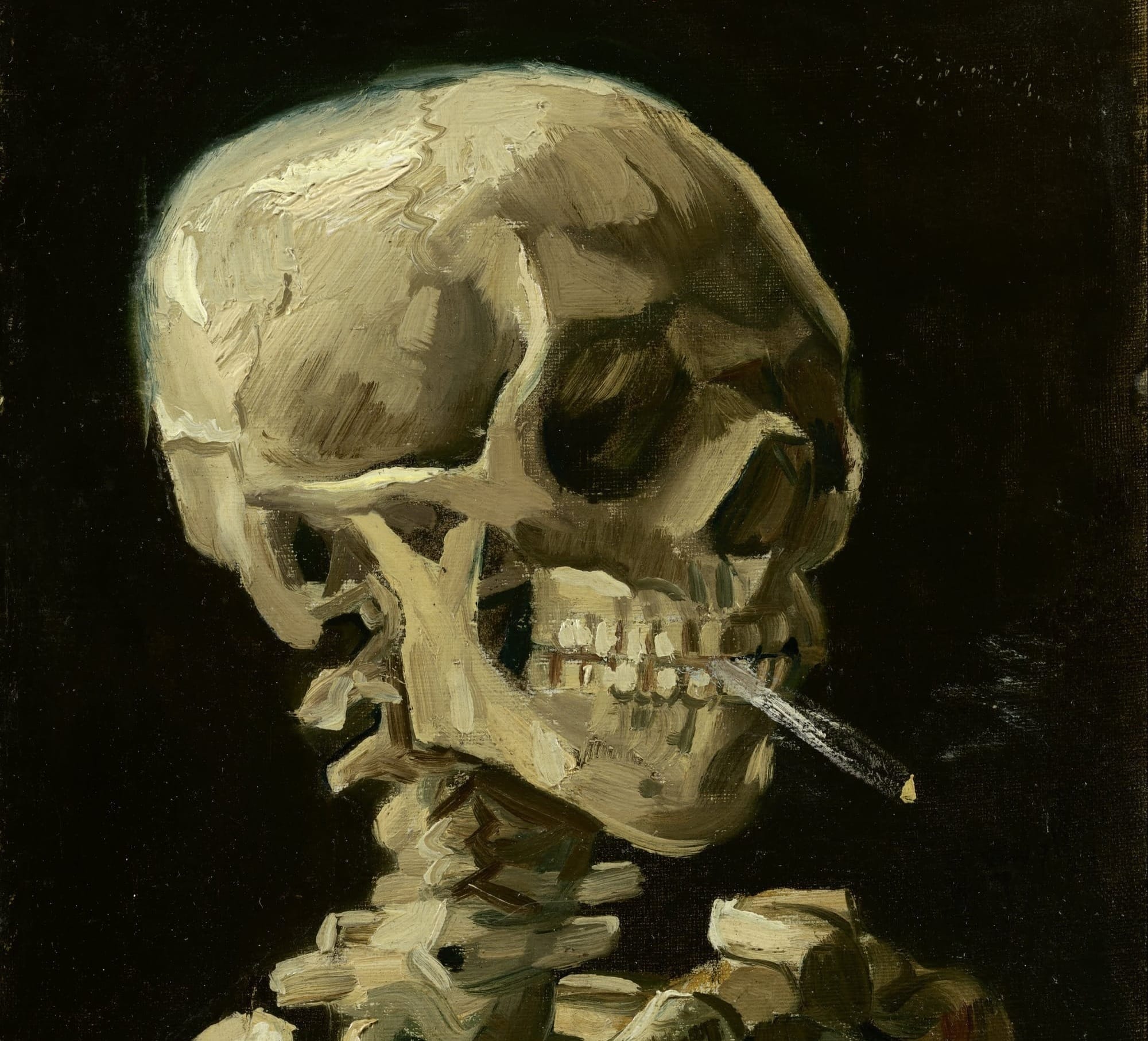
We previously added unified identities to the thirdweb SDK, allowing users to link multiple profiles as valid authentication options within your app. However, you couldn't tell which provider they had signed in with for the current session. In thirdweb v5.77.0, we've added the getLastAuthProvider
utility to the React module, allowing you to retrieve the most recently used provider from within any web context.
- Fixed a caching issue in our new headless components
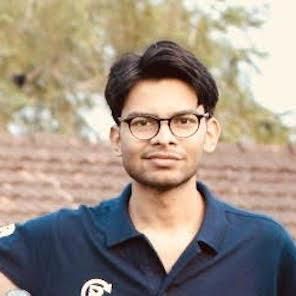
Users can now publish their contracts with contract references in deploy params.
What this means is, if there are address params in your contract's constructor, you can provide a reference to another published contract for those params. These reference contracts will then be deterministically deployed before deploying your contract.
You can specify these references in publish form as shown below:
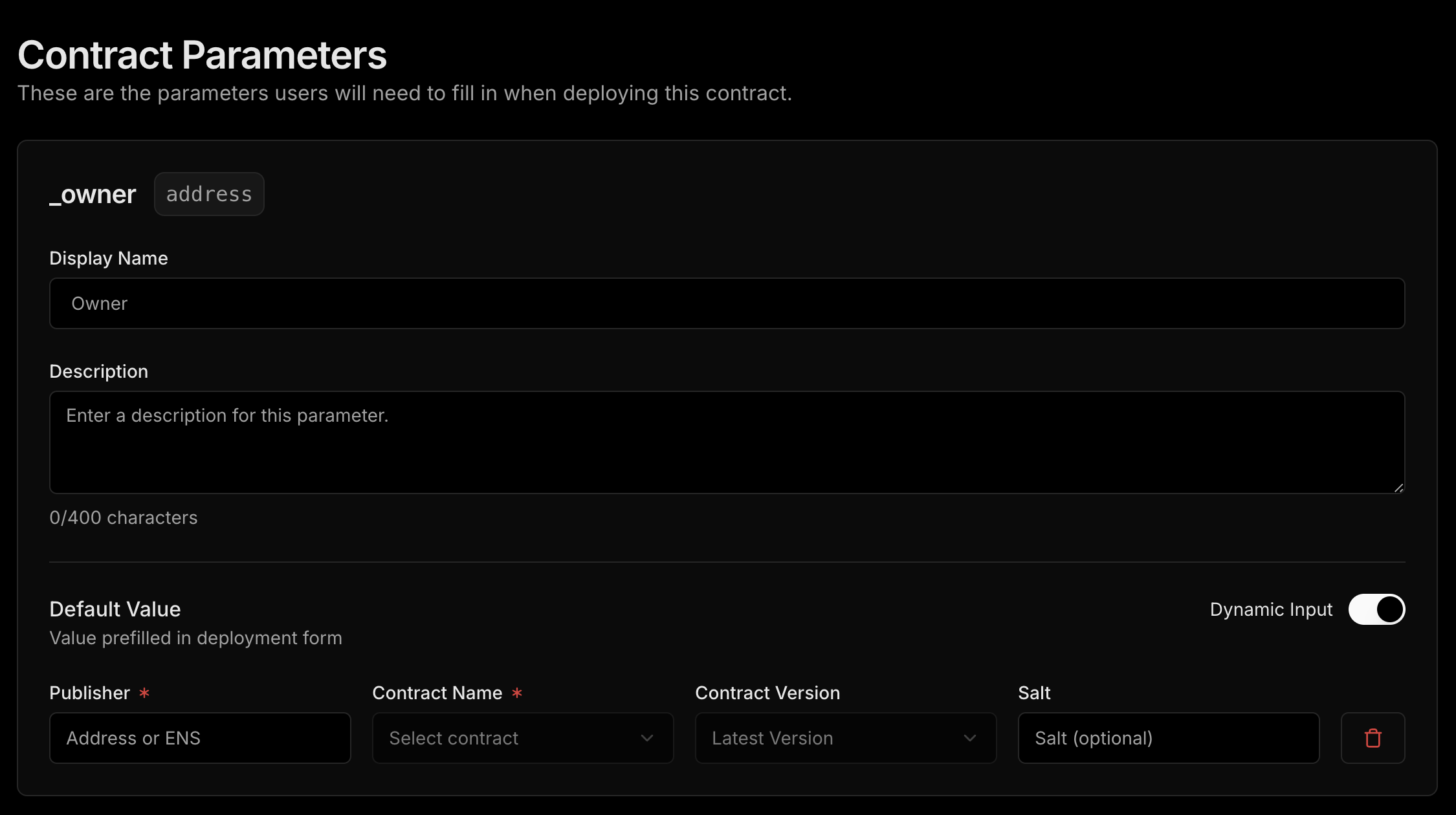
For e.g., _owner
param is of type address
and allows publisher to specify a contract reference. You can do this by selecting Dynamic Input
and providing details of the published contract. Please note that the contract being set as reference should be a published contract with default values pre-filled.
You can also setup a chain of such references, i.e. a referenced contract has another reference contract in its deploy params, and so on.
In addition to the address
type, you can also provide dynamic inputs for bytes
params. For e.g., if the bytes param is composed of address
, uint256
, and bool
, you can specify these values instead of giving an encoded bytes value. These will be encoded during deployment. The address param within this bytes param can again be a dynamic reference. See below:
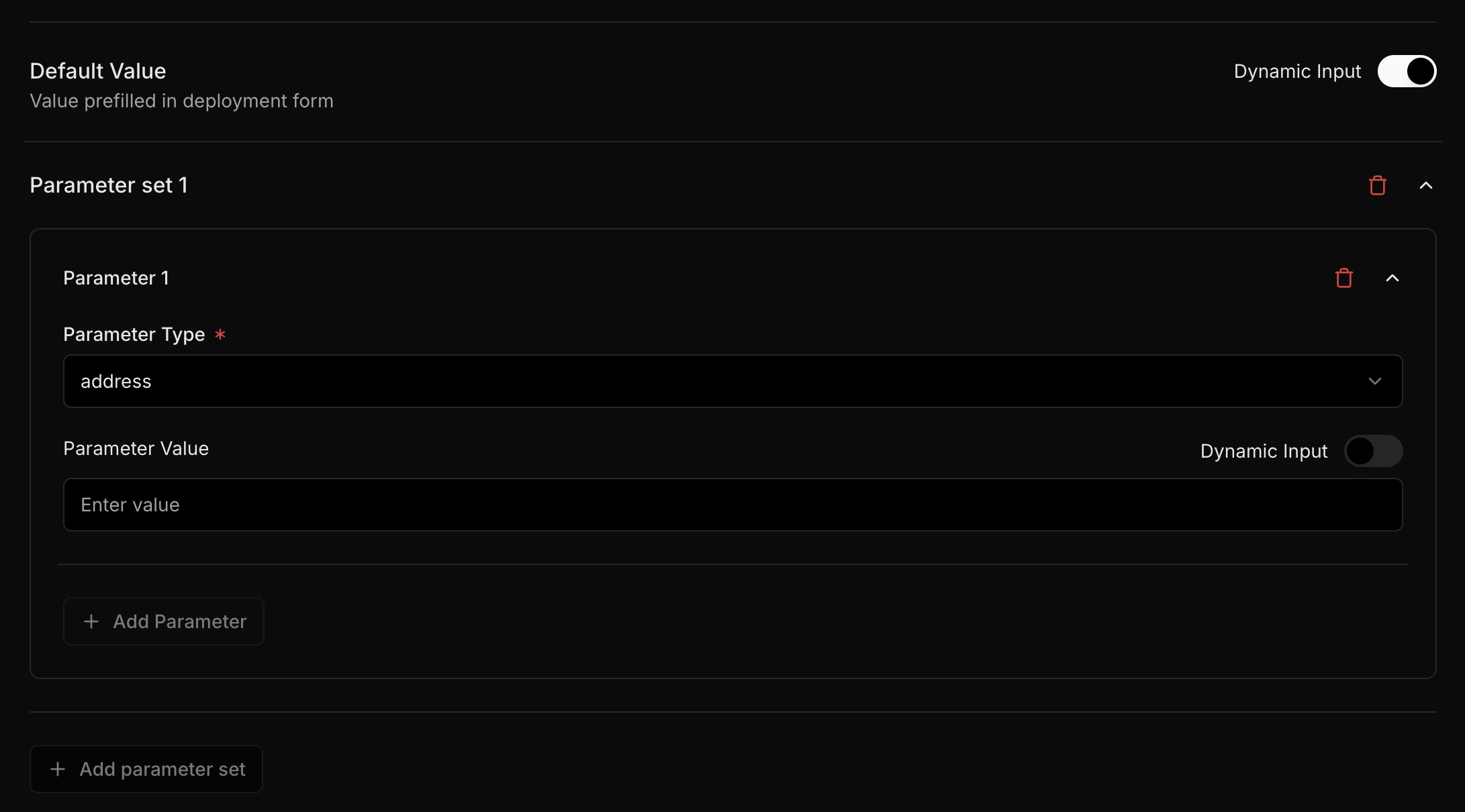
This feature works for address[]
and bytes[]
params too.
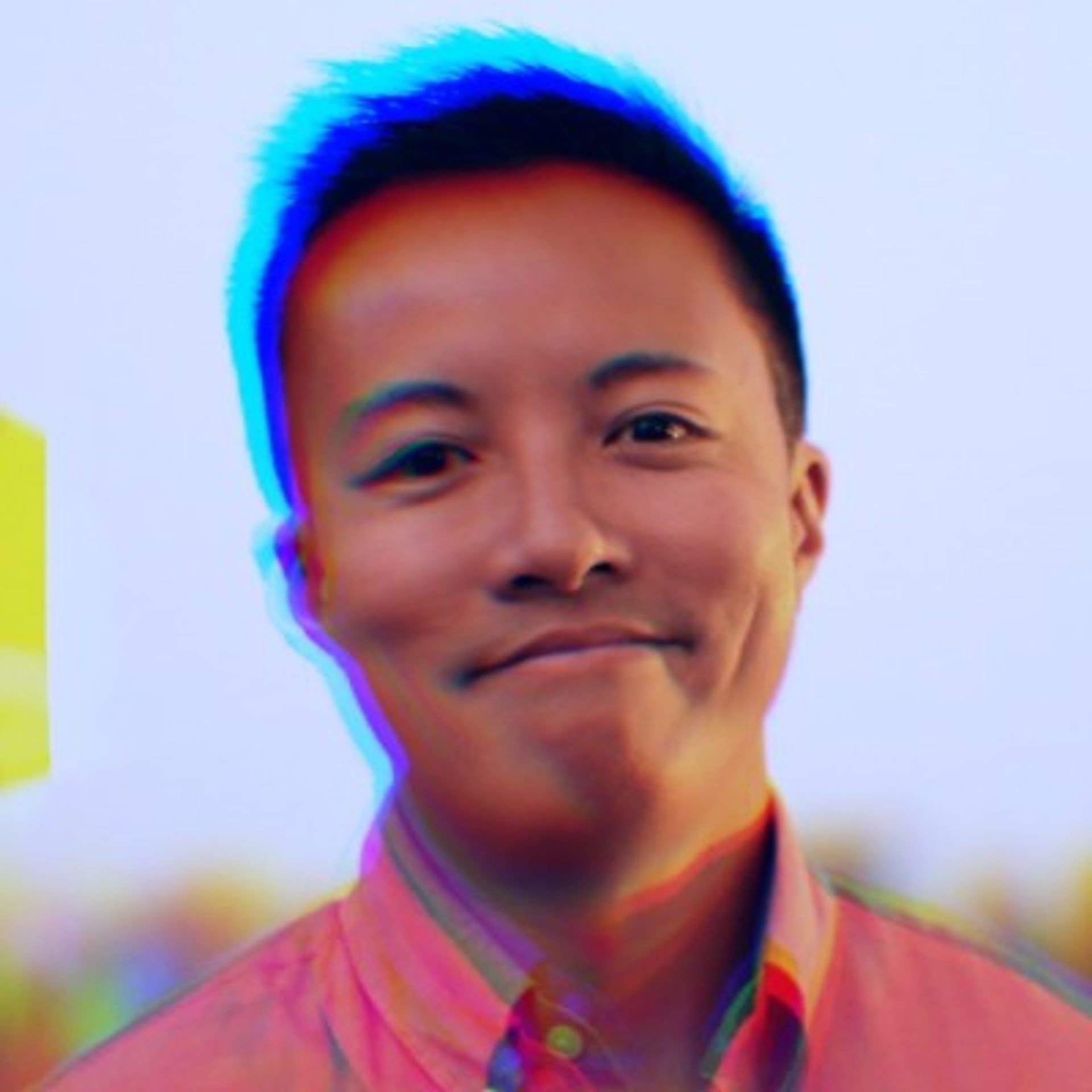
Engine is used by web2 and web3 companies for production use, powering 10M+ transactions per week. Making sure your Engine is secure and your funds are safe are critical, so here's security improvements we made (v2.1.20) and what's coming up.
We've completed our second code and infrastructure assessment from an independent third party auditor and will be sharing the report shortly.
No critical vulnerabilities were identified, and all reported issues were addressed.
- Improved CORS response handling. Clearer logs + responses if calls are made from invalid origins.
- Added modern browser security headers to restrict unused permissions (embedding, geolocation, camera, etc.).
- Updated dependencies to address publicly reported vulnerabilities.
singlePhaseDrop
added to allclaim-to
endpoints allowing users to set claim conditions and claim tokens in a single phase for custom drop contracts.gasPrice
can now be overridden allowing users to explicitly set the gas price for pre-EIP1559 chains.- Post-EIP1559 chains should continue to use
maxFeePerGas
andmaxPriorityFeePerGas
overrides, if needed.
- Post-EIP1559 chains should continue to use
- All
/mint-to
endpoints were updated to thirdweb v5 SDK and should have greatly improved response times. - Idempotency keys up to 200 characters are now supported.
- The codebase is updated with better organization and code standards, and we're enforcing linting rules moving forward.
The small team at thirdweb is on a mission to build the most intuitive and complete web3 platform. Our products empower over 70,000 developers each month including Shopify, AWS, Coinbase, Rarible, Animoca Brands, and InfiniGods.
See our open roles. We’d love to work with you!
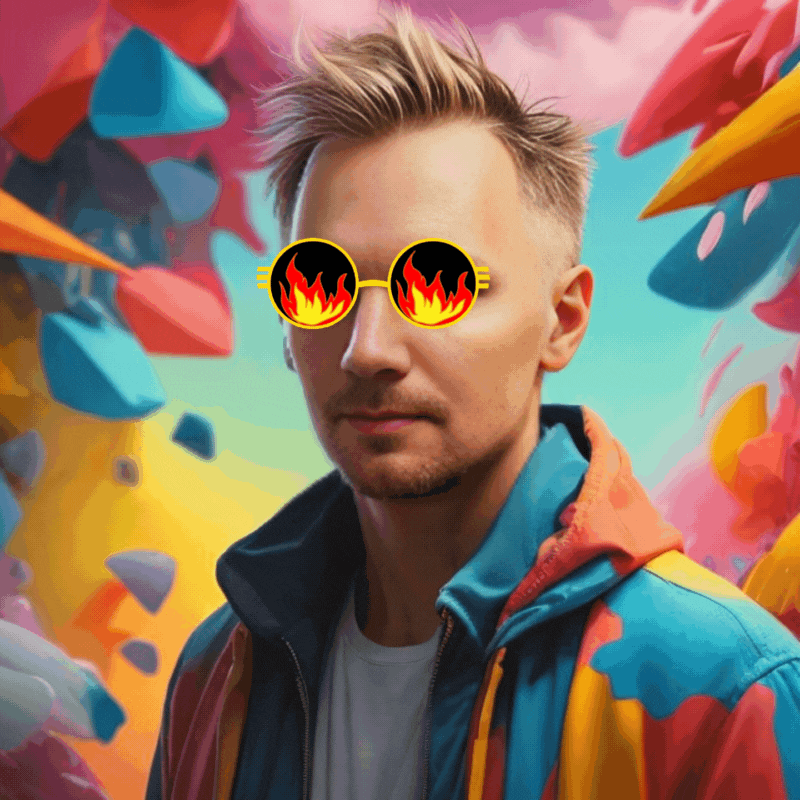
A new version of Insight is released, focusing on bringing decoded data to the API and bug fixes to the indexing logic.
Decoding for events and transactions in the API
Implemented functionality to decode event logs (supporting both indexed and non-indexed parameters) and transaction inputs whenever a query is made with. partial ABI.
For example, let's say we want to query all allocated withdrawal function calls on the TheCompact
contract (0x00000000000018DF021Ff2467dF97ff846E09f48
).
The partial ABI of the function is ((bytes,uint256,uint256,uint256,uint256,address))
, however the Insight API allows for named arguments, so we can use allocatedWithdrawal((bytes allocatorSignature,uint256 nonce,uint256 expires,uint256 id,uint256 amount,address recipient) _withdrawal)
We can do the following query to insight
and receive a response
The interesting part is the decodedData
property, which contains the decoded function input arguments
- Poller gap filling uses configured poll range - Introduced a limit on polling missing blocks, based on `blocksPerPoll` configuration instead of the whole missing range.
- Fixed signature calculation for partial ABI - Fixed a bug where various uint types in the partial ABI resulted in an incorrect signature
- Fixed nullpointer exception when checking if polling limit is reached
- Clickhouse delete performance - Updated to use lightweight deletes instead when handling reorgs, to reduce load on the DB
Learn more about Insight and how to use it.
Insight is open source.
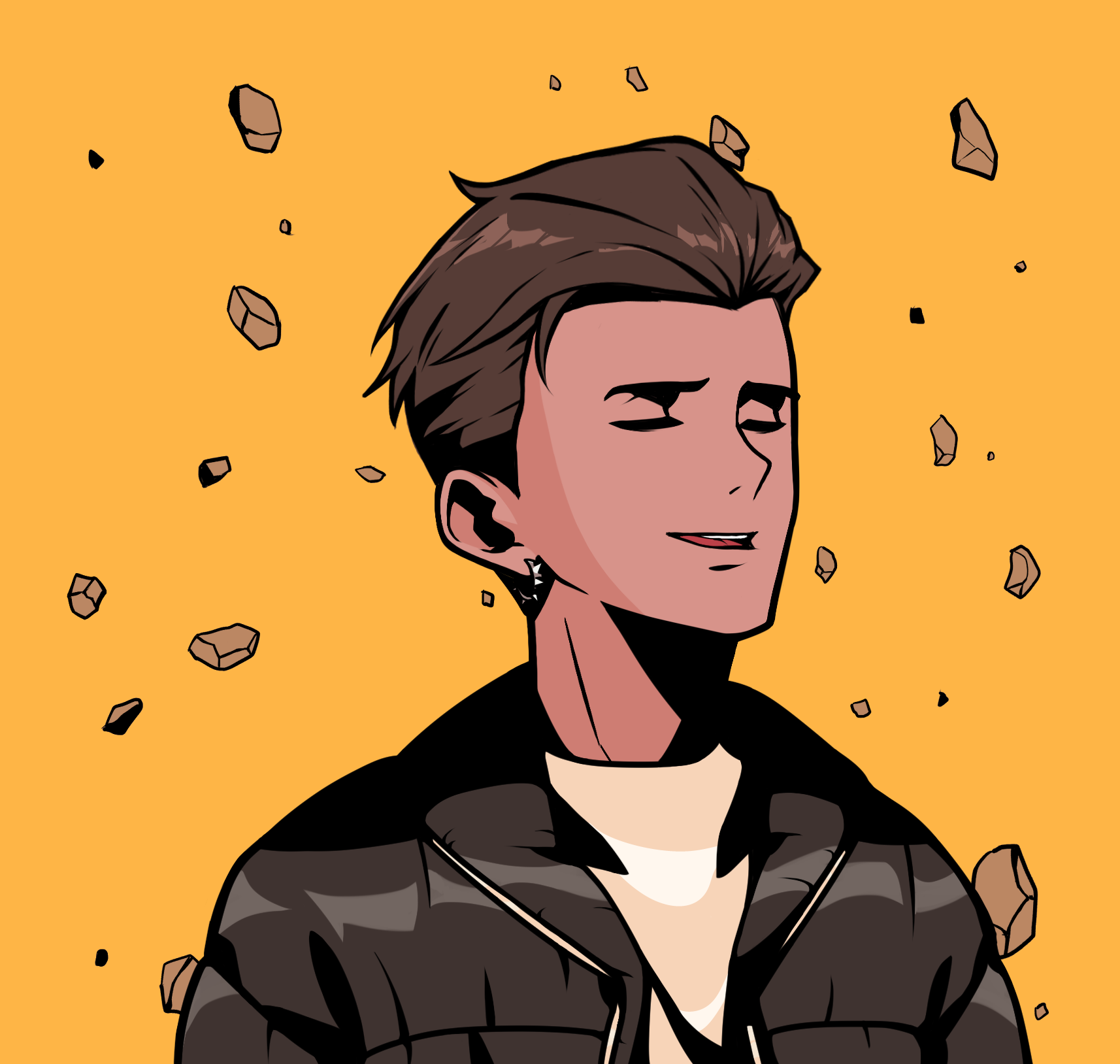
We've been iterating on our next generation smart accounts contracts. They are ERC-7579 compliant modular smart accounts, unlocking the growing catalog of account modules.
This is a BETA release, so expect some changes, but you can already start playing with it on both TypeScript and DotNet SDKs.
We have published 2 contracts to get you started, a modular account factory and a simple validator module.
A generic, un-opinionated smart account factory that lets you pick a smart account implementation and any default modules you like.
A simple validator module, with multi owner and session key support.
Once you have deployed both of those contracts, you can use them in the SDK to deploy accounts for you users, using the new Config
smart account presets.
You now have a modular smart account wallet instance that you can use with the rest of the SDK as usual.
You can also pass this configuration to the Connect UI components:
API and contracts are still in BETA - expect breaking changes and frequent updates.
Once stabilized, we will deploy a default factory and validator module on all chains which will be the default in the SDKs.
We're releasing this early so we can get some feedback from you, play with it on testnet and let us know what you think!
Happy building! 🛠️
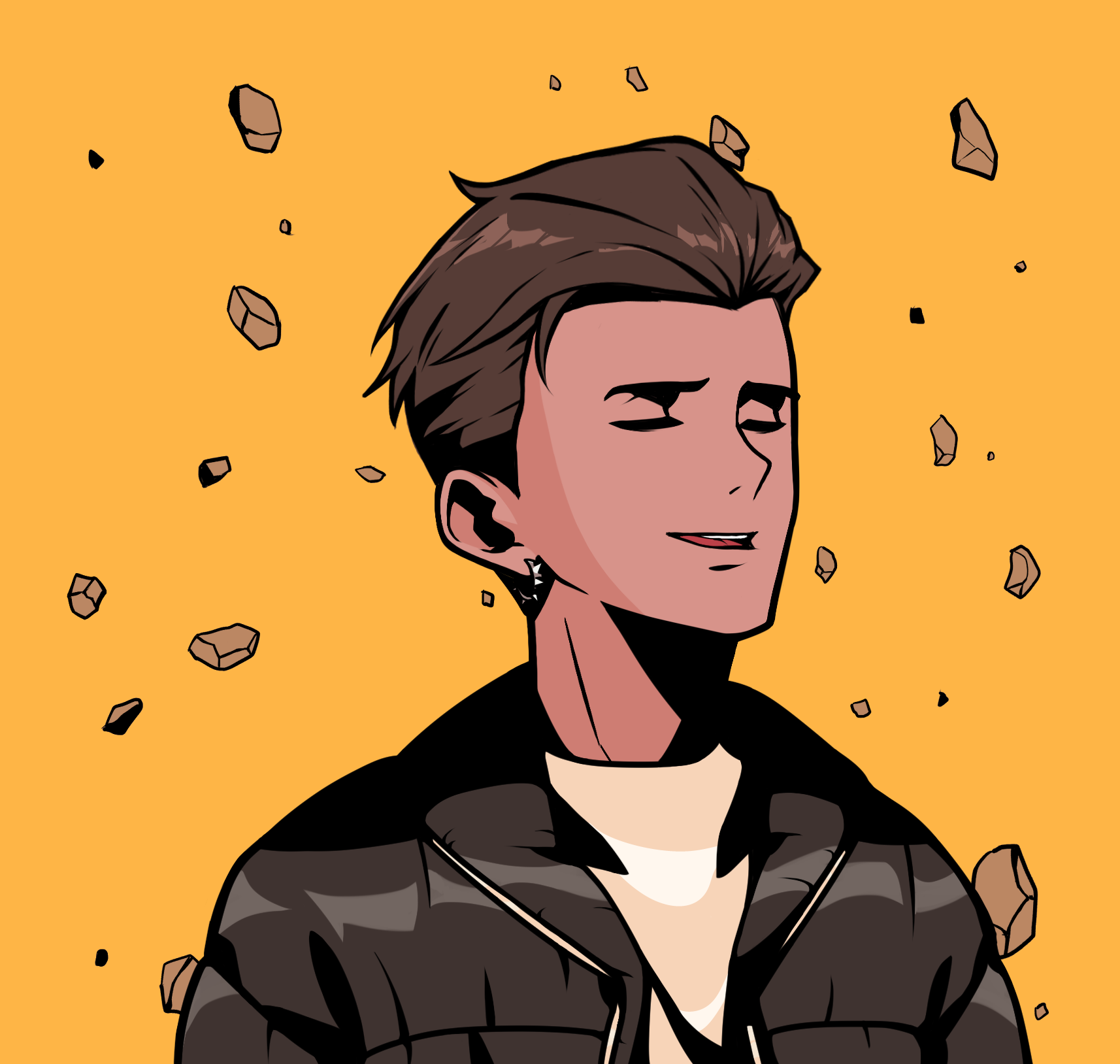
We just added support for ERC-6492 signatures in the TypeScript and DotNet SDKs. This means that you can now sign and verify signatures for smart accounts even if they haven't been deployed yet!
This massively improves the first time user flow when your app uses smart accounts and sign in with ethereum, no need to wait for the accounts to be deployed to proceed into the app the very first time.
It also reduces cost for app developers, since the cost of deploying smart accounts will only be paid on the first transaction, not when signing in.
No code changes for this one, just make sure you use the latest version of the SDK on both the signing and the verifying side, or any 6492 compatible signature verification library.
Happy building! 🛠️
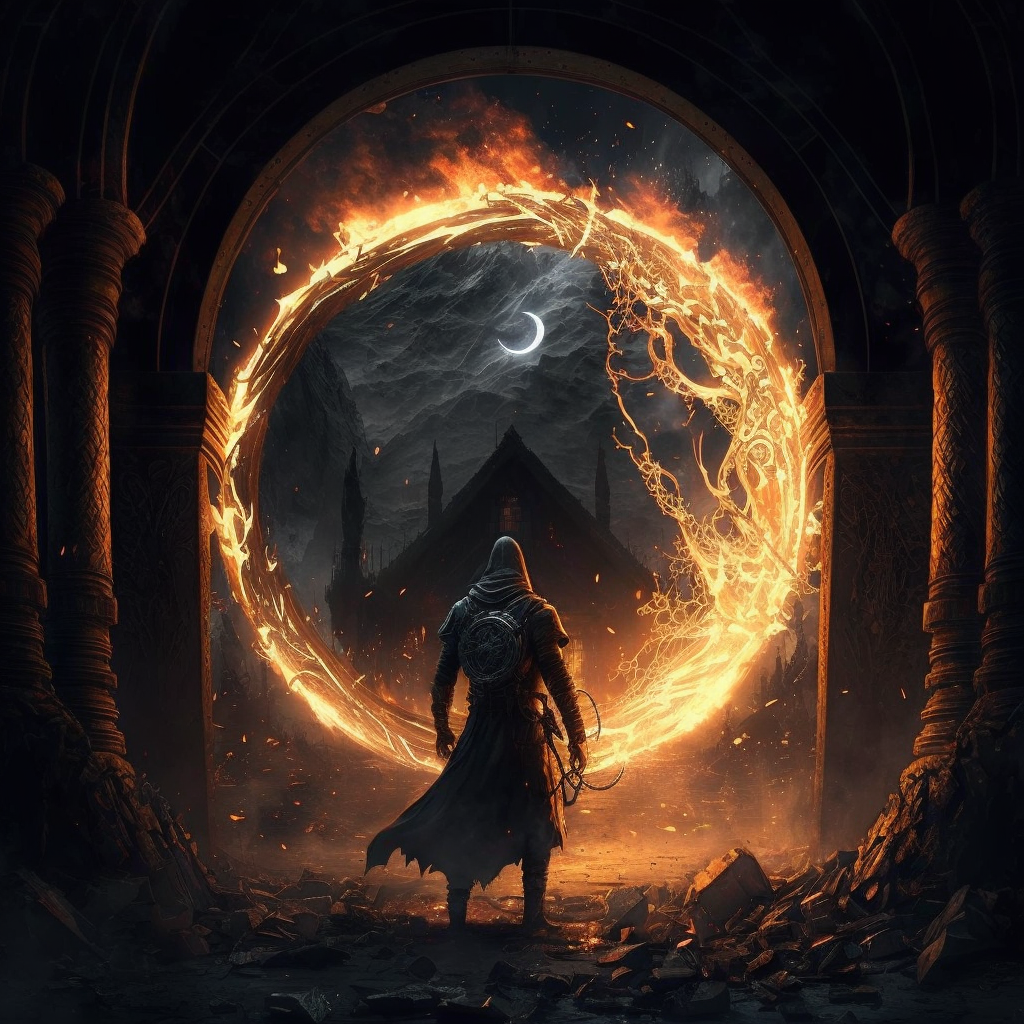
New chains: Appchain, Metal L2 Testnet, Metal L2, XSolla Testnet, Funki Sepolia, Sanko, Dos Testnet, Dos Mainnet, Bob, Bob Sepolia
We've added new chains to the platform this week!
Every chain comes out of the box with SDK support RPC, Engine and Account Abstraction capabilities. All you need is the chain id to get going.
For testnets, you'll also find a faucet in each chain page.
Happy building! 🛠️
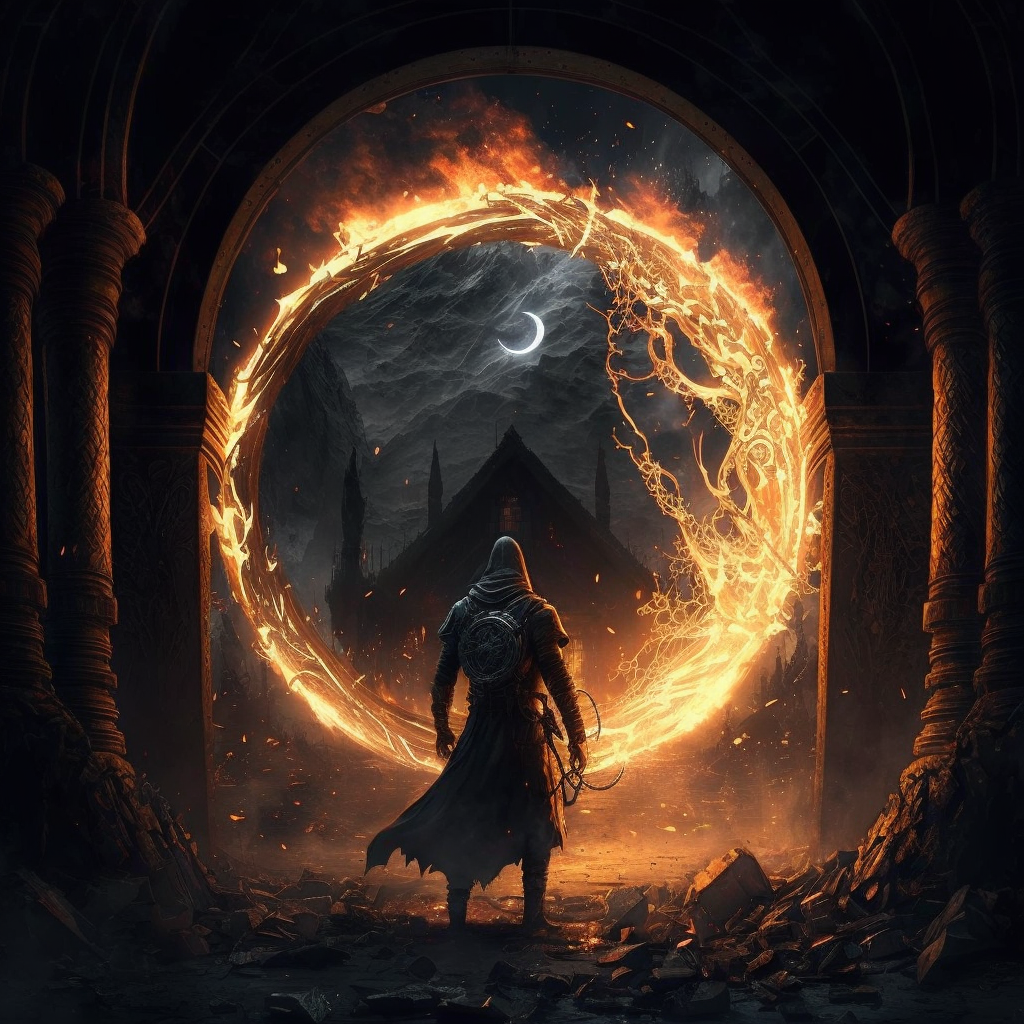
- Additions from Thirdweb's .NET SDK Release 2.11.0
SmartWallet.PersonalSign
no longer requires the SmartWallet to be deployed, increasing speed. Verification is done through ERC-6492. Validating a signature also works with ERC-6492 signatures now. Useful for apps that want to instantly authenticate (using Thirdweb Auth - SIWE for example) without incurring costs.purchaseData
can now be passed to Thirdweb Pay quote params, making Direct Payment flows fully featured.
InAppWalletOptions
now extendsEcosystemWalletOptions
(no changes to dx).- Slightly improved Playground UI.
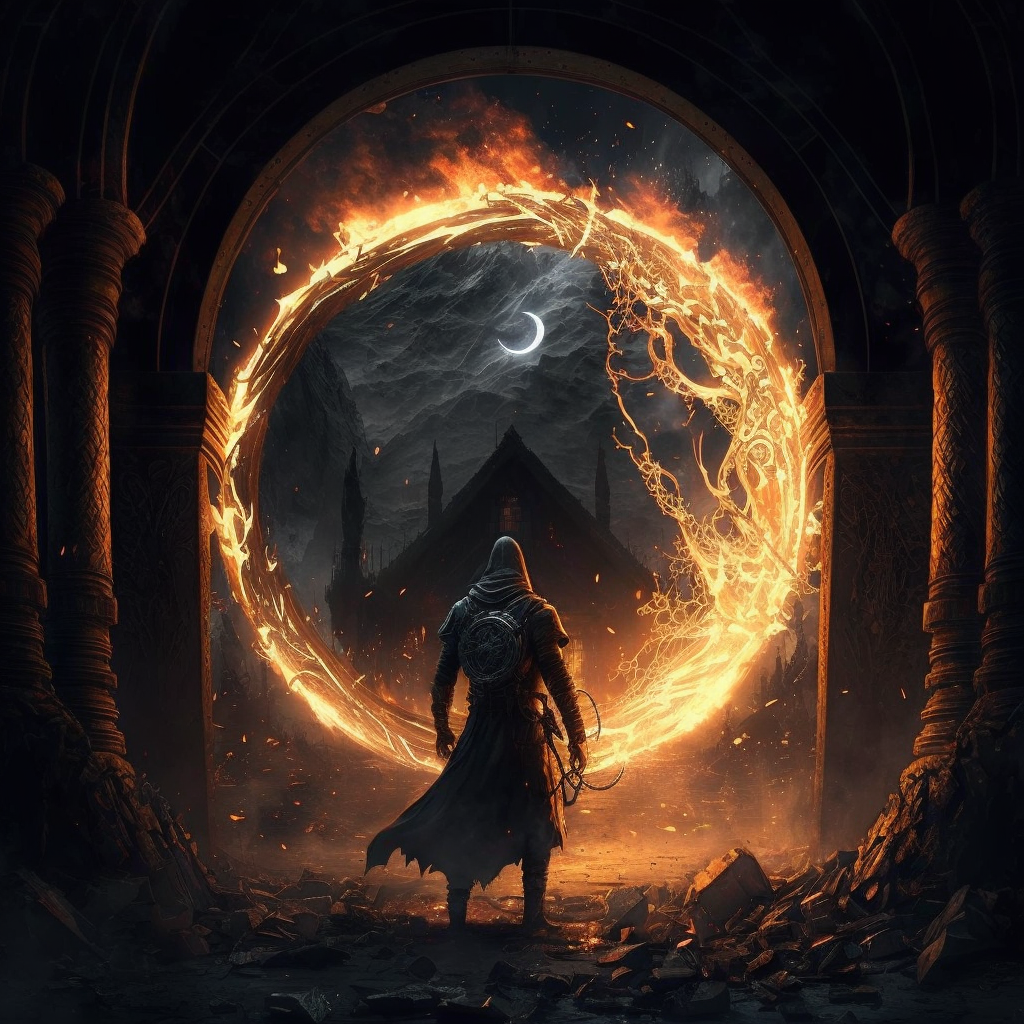
Added the ability to pay for gas with ERC20 tokens when using Smart Wallet.
- Currently supports Base USDC, Celo CUSD, and Lisk LSK tokens.
- Simply select a
TokenPaymaster
when creating aSmartWallet
and all transactions will be paid for with the corresponding ERC20 from the user's Smart Wallet. - Useful on L2s where the native token is ETH and you want to enshrine another coin or onramp your users directly to that coin.
- These TokenPaymasters entirely operate onchain, leveraging popular DEXes and decentralized price feeds from Uniswap & Chainlink.
- This product is in beta, and only compatible with EntryPoint v0.7.0, which we auto select for you if not overriden when configurin your wallet.
Example:
The latest update brings unparalleled marketplace integration to your fingertips! All 36 marketplace engine calls are now available in C++, Blueprints, and as async tasks, complete with supporting structs to streamline your workflow. Dive into enhanced examples, fetch IPFS nodes seamlessly, and enjoy compatibility with Unreal Engine 5.2 and 5.5 for even greater flexibility. We've cleaned up runtime settings, optimized dynamic tasks, and resolved key build errors to ensure a smooth development experience. Get ready to unlock the full potential of your marketplace projects with these powerful new tools!
- Bug Report and Feature Request Templates in Repo
- Missing C++ functions for exising engine calls
- All 36 marketplace engine calls in c++, Blueprints, and as async tasks with supporting structs
- Sign In With Ethereum and Link with SIWE
- Fetch IPFS nodes
- QR Code Generator for future use
- Unbind Route on destroy for Browser
- UE_5.2 compatibility for customer
- UE_5.5 compatibility
- Cleanup Runtime settings
- Cleanup duplicate async tasks for dynamic tasks
- Updated examples
- Updated Rust libs
- intrinsic build errors
- OAuthBrowserWidget Error being empty
- Moved BuildPlugin to its own repo
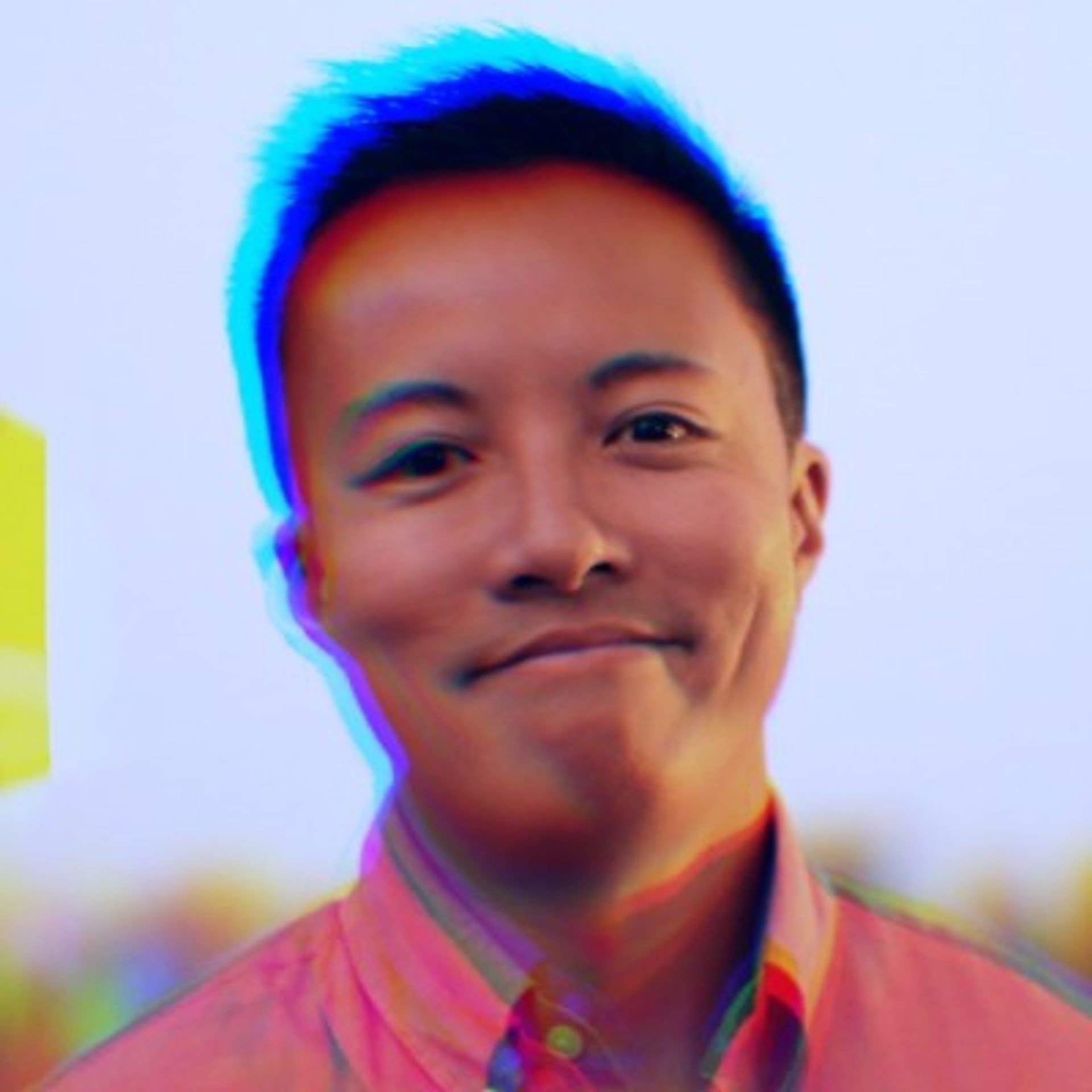
Since introducing Smart Backend Wallets we introduced some new capabilities to Engine and over a dozen bugfixes.
It is important to verify the signature header for Engine webhook calls to verify they came from your Engine. The dashboard now allows you to send a test payload to determine if your webhook endpoint is unreachable, not handling the webhook signature properly, or returning a unexpected response.
On the Webhooks dashboard page, select ... > Test webhook to send an example webhook payload to your backend and see the status code and response body.
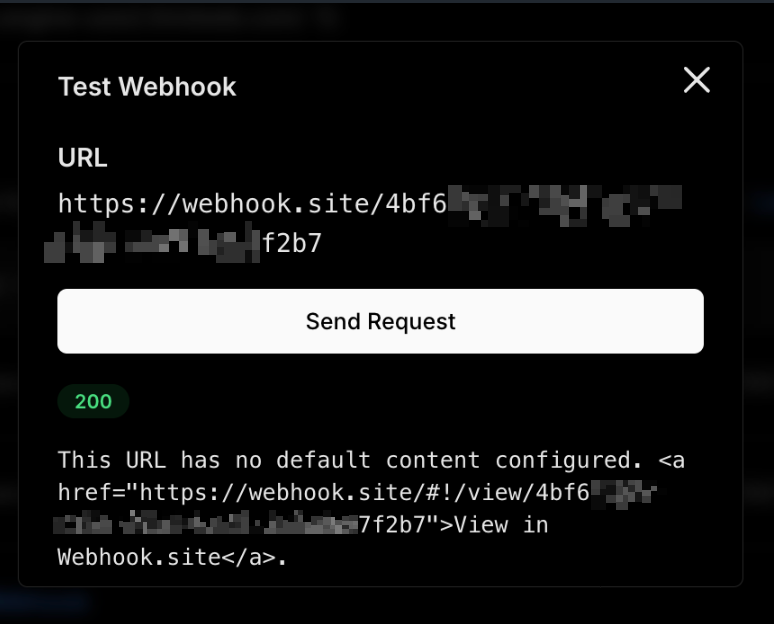
You can now delete backend wallets directly from the dashboard. Keep your backend wallets list tidy by removing unused backend wallets.
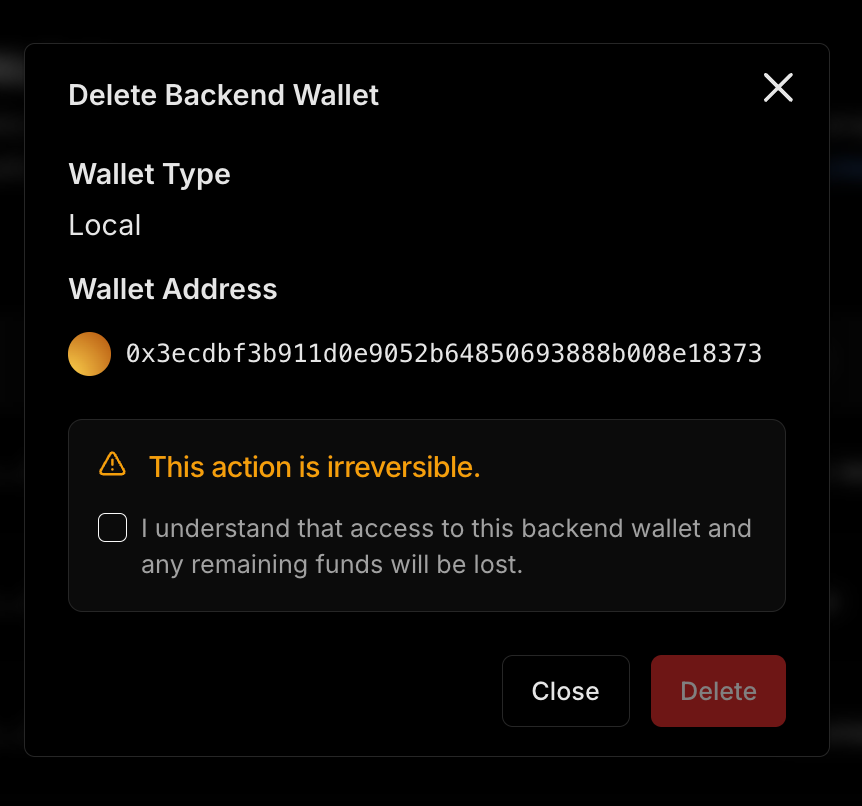
Engine now estimates the required amount of gas when it attempts to send a transaction without sufficient gas funds:
Insufficient funds in 0x4Ff9aa707AE1eAeb40E581DF2cf4e14AffcC553d on Polygon Mainnet. Transaction requires > 0.000682043142837 POL.
Lastly we've been squashing bugs to ensure your Engine handles transactions as reliably as possible.
- Fixed Contract Subscriptions to support all chain IDs (previous large chain IDs like Xai Sepolia were unsupported).
- Updated dependency libraries to address reported vulnerabilities.
- Fixed malformed transactions causing the transactions to not load in the dashboard.
- Fixed an edge case where errors for read contract calls were dropped.
- Fixed an edge case in nonce self-healing.
- Fixed Smart Backend Wallet support for certain extension endpoints.
- Handled a different RPC response that could cause a nonce to be skipped.
- See Engine release notes for more details.
The small team at thirdweb is on a mission to build the most intuitive and complete web3 platform. Our products empower over 70,000 developers each month including Shopify, AWS, Coinbase, Rarible, Animoca Brands, and InfiniGods.
See our open roles. We’d love to work with you!
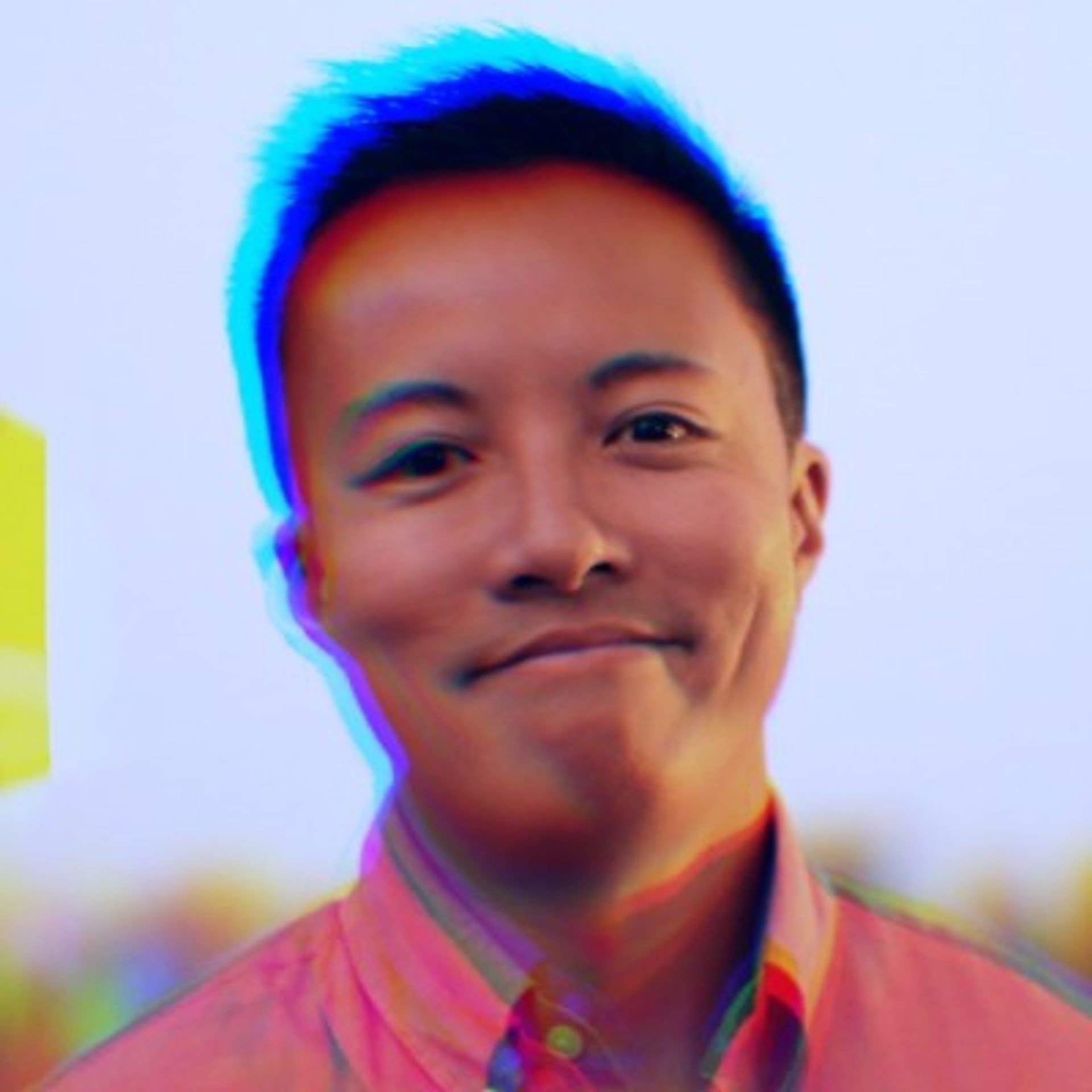
The Engine dashboard now shows all recent versions with timestamps and allows you to select any version to upgrade or downgrade to.
Find the improved version selector in the upper right corner of the Engine dashboard.
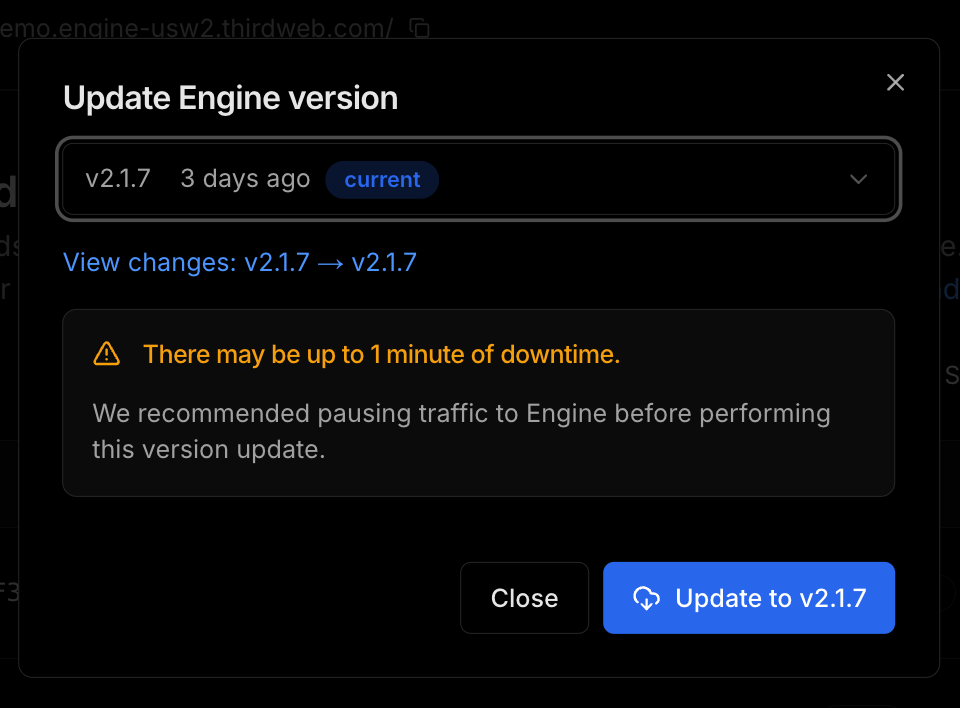
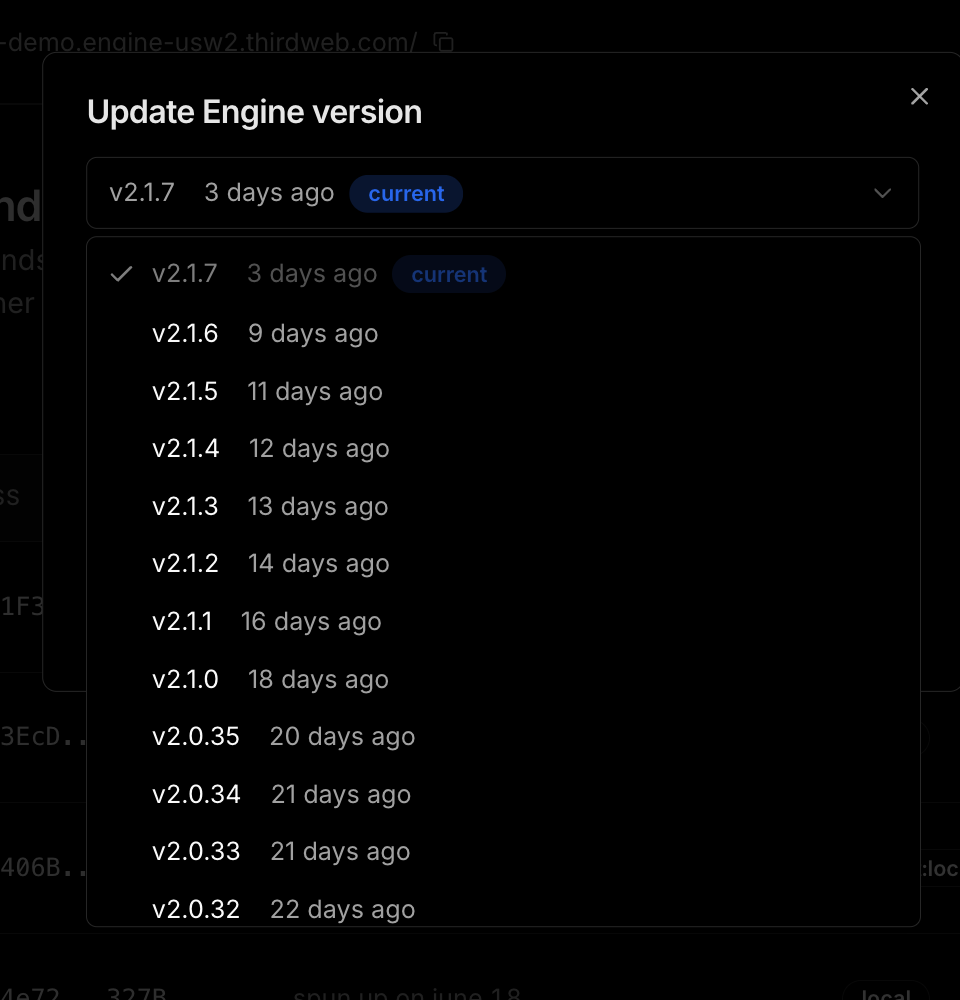
While Engine releases follows semantic versioning, non-major updates may add input validation or fix an incorrect response (e.g. a 500 that should have been a 400 response). These fixes correct behavior, but in rare cases this may conflict with how your backend sends or parses requests. In these cases, you can now self-service downgrade to a previous Engine version.
Example: Engine used to rely on an older SDK that did not strictly enforce ABIs. In a newer update, provided ABIs must match the proper spec. This may cause write calls that provided an incomplete ABI to start throwing an error.
We're aiming to provide visibility in the dashboard when Engine versions change shortly. We also plan to roll out a predictable schedule when cloud-hosted Engines may receive critical security or reliability updates.
The small team at thirdweb is on a mission to build the most intuitive and complete web3 platform. Our products empower over 70,000 developers each month including Shopify, AWS, Coinbase, Rarible, Animoca Brands, and InfiniGods.
See our open roles. We’d love to work with you!
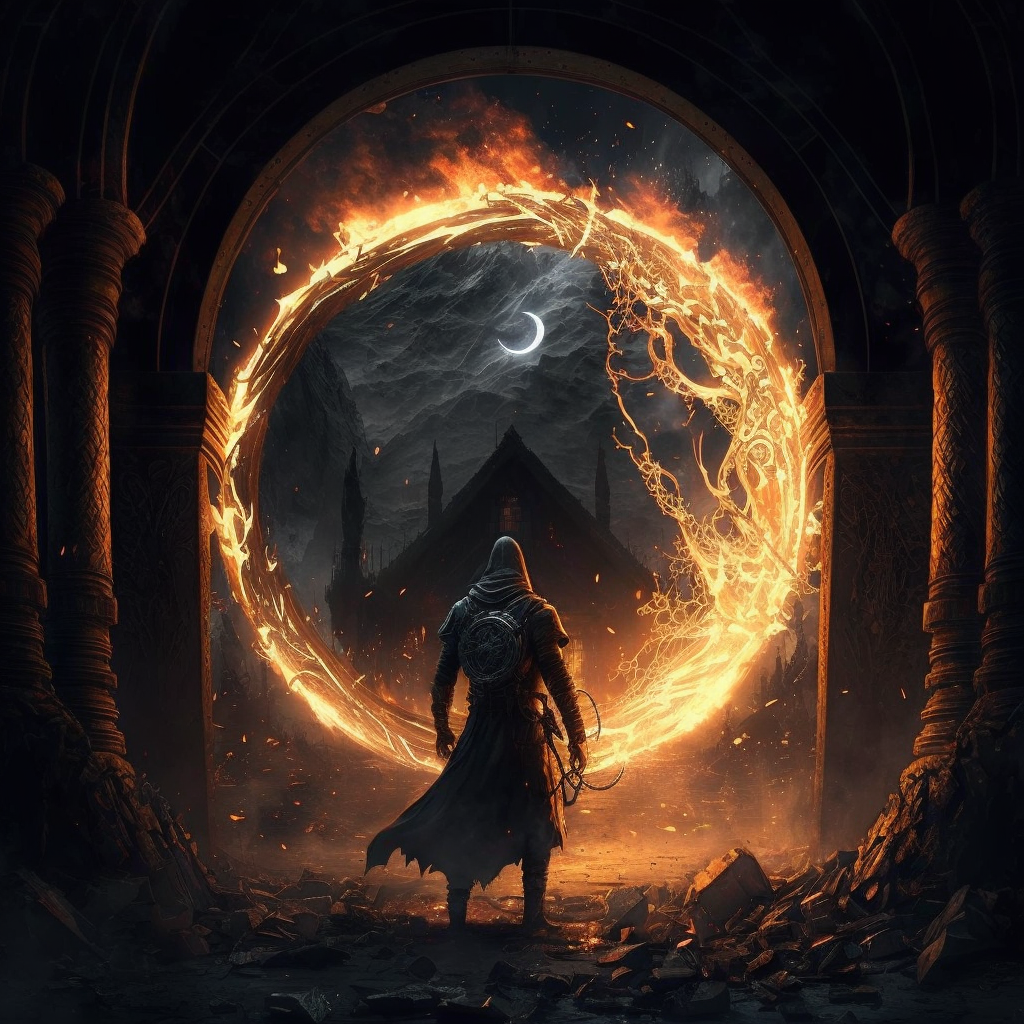
- Added
AuthProvider.Steam
as a login option for In-App or Ecosystem Wallets.
- Added the ability to pay for gas with ERC20 tokens when using Smart Wallet.
- Currently supports Base USDC, Celo CUSD, and Lisk LSK tokens.
- Simply select a
TokenPaymaster
when creating aSmartWallet
and all transactions will be paid for with the corresponding ERC20 from the user's Smart Wallet. - Useful on L2s where the native token is ETH and you want to enshrine another coin or onramp your users directly to that coin.
- These TokenPaymasters entirely operate onchain, leveraging popular DEXes and decentralized price feeds from Uniswap & Chainlink.
- This product is in beta, and only compatible with EntryPoint v0.7.0, which we auto select for you if not overriden when configurin your wallet.
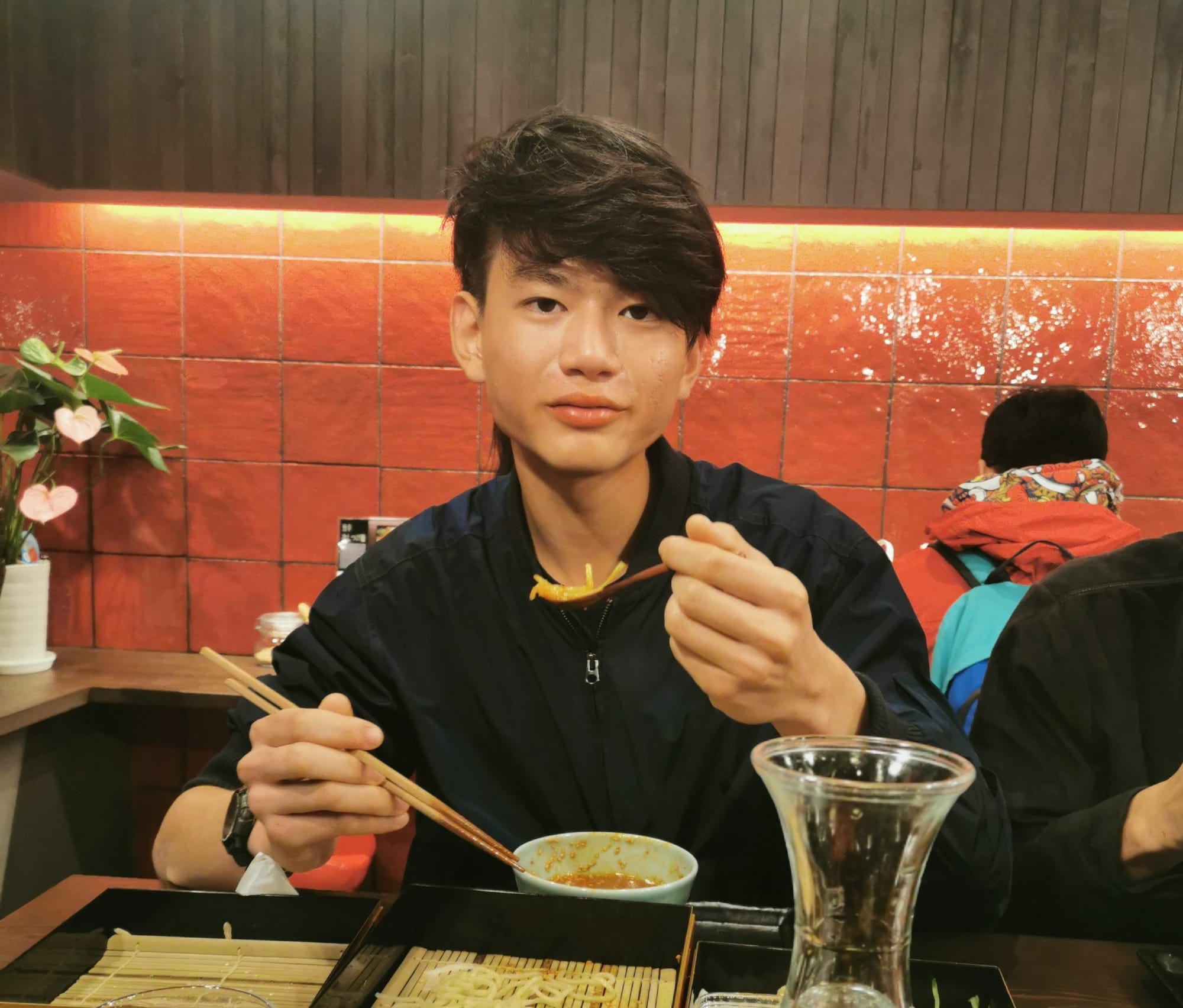
We supported pregeneration for enclave wallets a while back.
Today, wallet pregeneration is available for in-app wallets too!
What does this mean?
This means that you can now know the in-app wallet address of a user before they register on your platform!
This allows you to perform airdrop, pre-load certain assets, and more!
The API is the same as ecosystem wallets except we don't have to include the ecosystem details:
On top of that, we also now support pregeneration via custom IDs. This means that you can know your user's address ahead of time even if you use a custom authentication methods like JWT or an auth endpoint.
Here's what that would look like:
For more information and background on what pregeneration is all about, check out the newly updated documentation here.
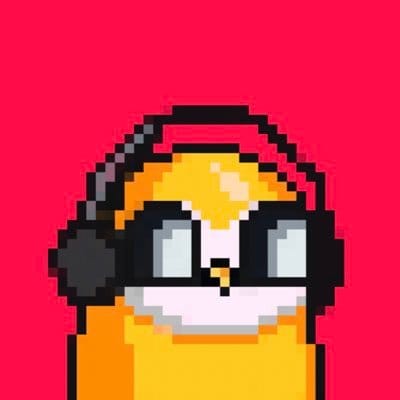
We're excited to announce that thirdweb Insight has expanded its blockchain data querying capabilities with support for six new chains:
- Donatuz
- Avalanche
- Soneium Minato
- Fhenix- Helium
- Ape Chain
What This Means For Developers
With these additions, you can now use all of Insight's powerful features across these new chains:
- Query on-chain events with the Events Blueprint
- Track transactions with the Transactions Blueprint
- Monitor token balances (ERC-20, ERC-721, ERC-1155) with the Tokens Blueprint
https://<chain-id>.insight.thirdweb.com
Replace `<chain-id>` with the specific chain ID you want to query.
For example, to query Avalanche data:
Use Cases
These new chain additions enable various use cases across different sectors:
- DeFi
- Track token movements and trading volume across new DeFi ecosystems
- Monitor liquidity pools and DEX activity
- Analyze cross-chain token economics
- Gaming
- Track in-game assets and NFTs on gaming-focused chains
- Monitor player rewards and achievements
- Analyze gaming economy metrics
- NFTs
- Track NFT collections and transfers across new marketplaces
- Monitor NFT trading activity and ownership
- Analyze collection performance metrics
View All Supported Chains
For a complete list of supported chains and their respective chain IDs, check the thirdweb chainlist.
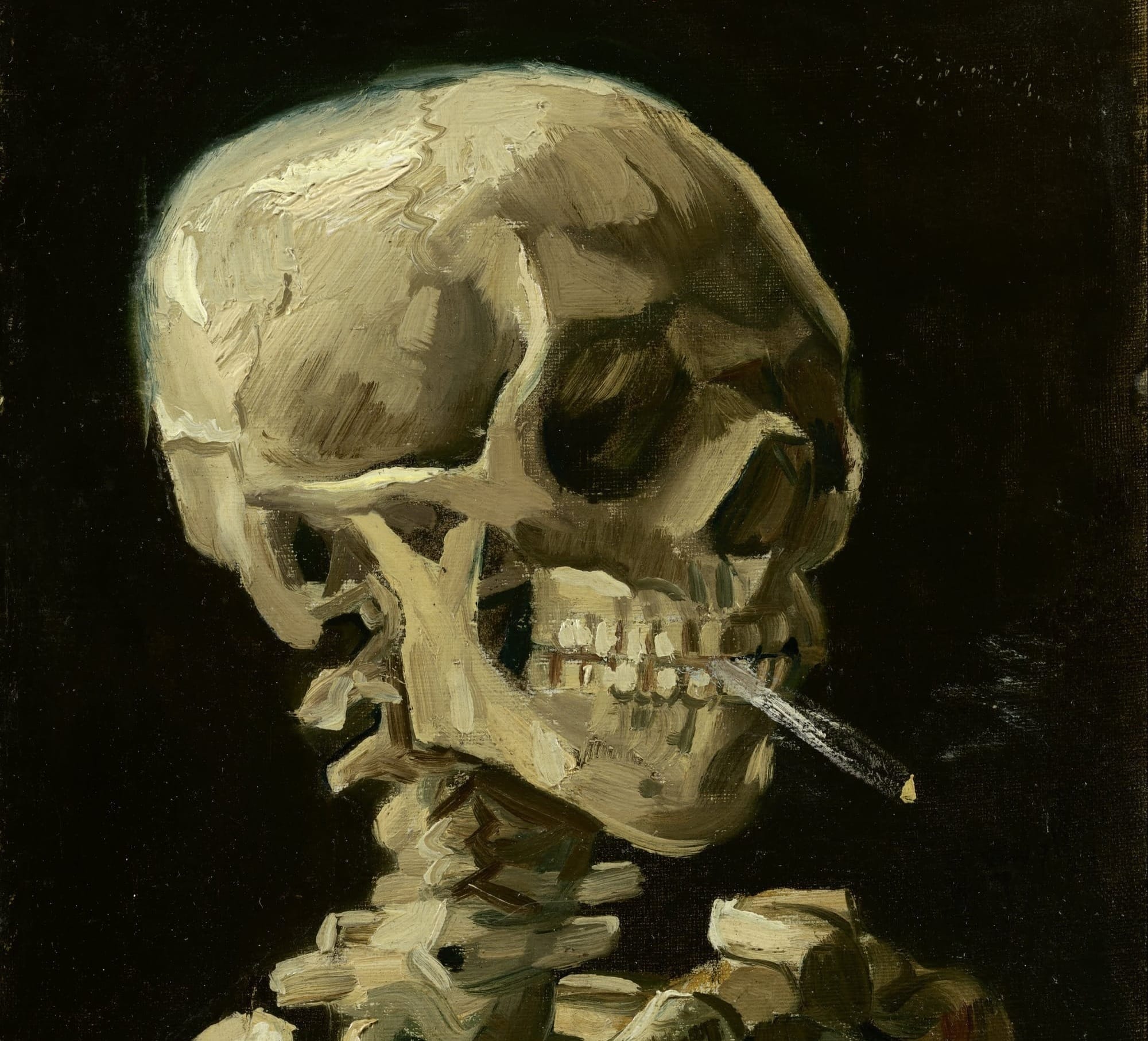
As of v5.69.0, we've added Steam as an authentication option in our TypeScript SDK for all the games and game enjoyooors out there. You can setup Steam auth just like any other authentication method.
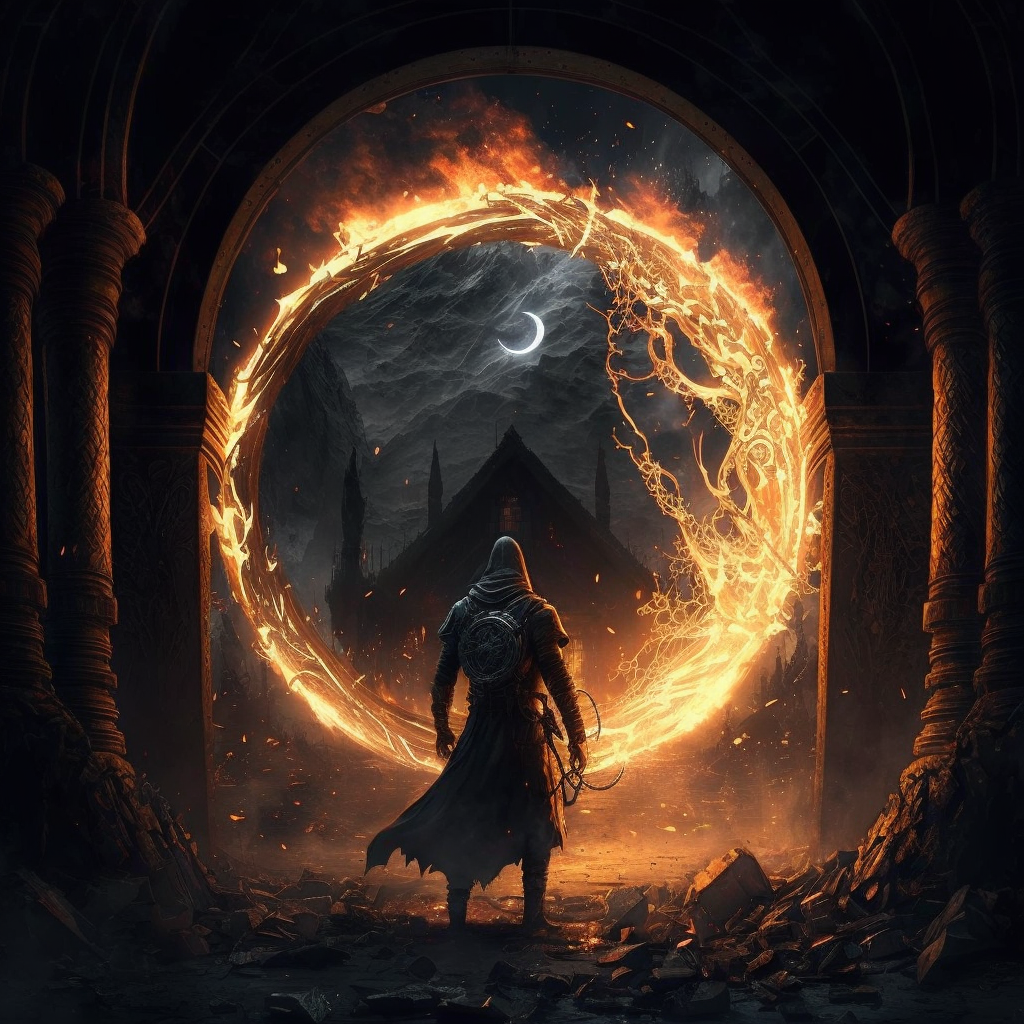
New chains: Etherlink, Etherlink Testnet, Lisk Mainnet, Zero Mainnet, Cronos zkEVM Testnet, Cronos zkEVM
We've added new chains to the platform this week!
Every chain comes out of the box with SDK support RPC, Engine and Account Abstraction capabilities. All you need is the chain id to get going.
For testnets, you'll also find a faucet in each chain page.
Happy building! 🛠️
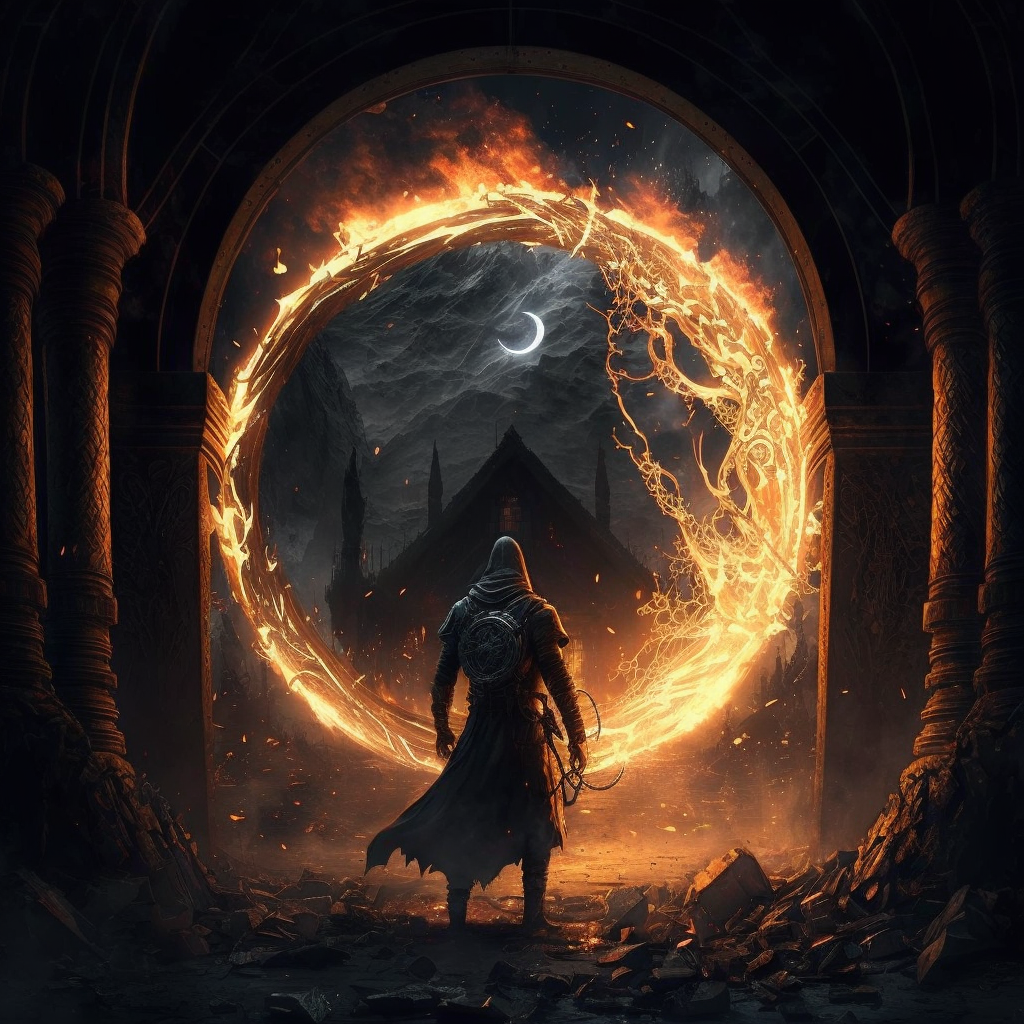
- Fixed an issue with typed data signing using In-App/Ecosystem Wallets when one of the fields was larger than Uint32.
- Improved NFT Metadata Population & Added Filters
- The
ERC1155_GetOwnedNFTs
extension now returns theQuantityOwned
metadata field correctly for each NFT. - The
ERC721_GetNFT
andERC721_GetAllNFTs
extensions can now take in a newfillOwner
parameter that defaults to true and decides whether to make additional requests to populate theOwner
field of the metadata, otherwise defaulting toConstants.ADDRESS_ZERO
. - The
ERC1155_GetNFT
andERC1155_GetAllNFTs
extensions can now take in a newfillSupply
parameter that defaults to true and decides whether to make additional requests to populate theSupply
field of the metadata, otherwise defaulting toBigInteger.MinusOne
.
- The